Creating Stunning Geometric Patterns with Python's Turtle Module
Written on
Introduction to Animated Geometric Patterns
This guide will walk you through the process of crafting geometric patterns with Python's Turtle library.
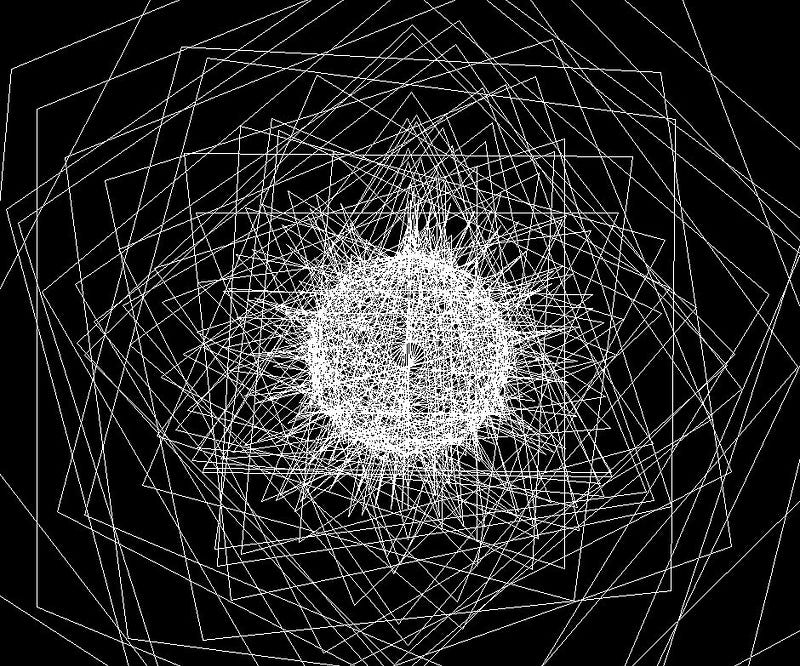
The Turtle module is an integrated library in Python designed for creating shapes, animations, and simple games on a canvas. Originally introduced in 1967 as a teaching tool, it remains a popular choice for educational programming. Because it comes pre-installed with Python, you can easily start using it by importing the necessary components directly into your project:
from turtle import Turtle, Screen
This command imports the 'Turtle' and 'Screen' sub-modules, allowing you to create graphical content.
Setting Up the Drawing Environment
To configure your drawing environment, you'll need to set up the screen and the turtle pen. Here's a basic configuration:
s = Screen()
s.bgcolor("black")
s.title('More Than Just a Circle')
By default, the turtle screen initializes with a white background. In this example, we want a black backdrop with a white drawing pen:
sketch_pen = Turtle()
sketch_pen.pencolor("white")
sketch_pen.speed(0)
sketch_pen.pensize(1)
The pensize function adjusts the pen's thickness, while pencolor sets its color. For a full range of colors and their corresponding turtle names, you can refer to the official documentation. You can also modify the drawing speed, with the option to set it to the fastest mode:
sketch_pen.speed("fastest")
With the turtle screen and pen configured, let’s move on to creating some captivating abstract geometric designs.
Understanding Drawing Commands
To create patterns, you'll use specific commands to control the turtle's movement on the screen. Here are a few essential commands:
- forward (distance): Moves the turtle forward by the specified distance.
- back (distance): Moves the turtle backward by the specified distance.
- right (angle): Rotates the turtle to the right by the given angle.
- left (angle): Rotates the turtle to the left by the given angle.
- goto (x, y=None): Moves the turtle to a specified coordinate on the screen.
The core logic of our code is outlined as follows:
length, angle = 0, 0
sketch_pen.penup()
sketch_pen.goto(0, 100)
sketch_pen.pendown()
while True:
sketch_pen.forward(length)
sketch_pen.right(angle)
length += 1
angle += 0.5
if angle == 270:
break
This code snippet initializes length and angle to zero. The penup(), goto(), and pendown() commands position the pen at a specific starting point. The while loop continues indefinitely until the angle reaches 270 degrees, at which point it stops. During this loop, the turtle moves forward by an increasing length and turns right by an increasing angle, creating dynamic geometric shapes.
To see the result of this code, check out the animated GIF representation of an abstract geometric pattern generated using Python's Turtle module.
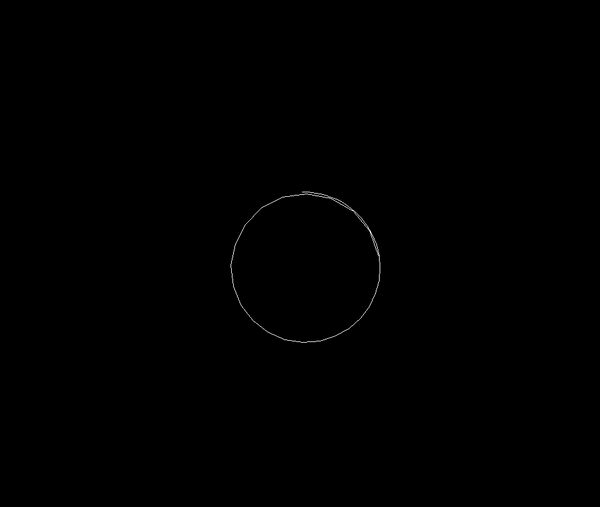
You can copy the code provided in this article into a .py file and run it to generate an animation similar to the one shown above. Have fun exploring!
Explore More with Video Tutorials
The first video, "Create Mind-blowing Geometric Shape Pattern Animations | After Effects Tutorial," showcases techniques to craft stunning geometric animations.
The second video, "Geometric Shape Animation in After Effects || Project Breakdown," provides a detailed breakdown of creating geometric animations using After Effects.
Conclusion
Thank you for reading! If you found this guide helpful, please feel free to show your support by clapping, sharing, commenting, or reaching out to me directly.
References: